5 ways to edit EnergyPlus input files
I've created an EnergyPlus input file, but how do I edit it? Do I have to edit it manually? Can I using programming to create many variants of my base-case file? This post answers these questions.
At the time of writing, and based on EnergyPlus version 9.6.0, there are two possible input data formats. The Input Data File (idf) format is the older version which has been used for many years. The EnergyPlus JavaScript Object Notation (epJSON) format is the newer version introduced a few years ago. Both formats contains exactly the same information, describing the building inputs and modelling parameters, and the only difference is that they store this information in different ways.
What are idf files?
idf files are standard text files which can be opened with text editors (such as the Notepad app on Windows). The text is structured in a particular format unique to EnergyPlus. In essence this is a list of input objects, where each object has a number of parameters (or fields) associated with it. This presents a challenge to working with idf files as this non-standard format means that general programming languages will not have standard parsers for working with these files. Below is an excerpt from the '1ZoneUncontrolled.idf' file in the ExampleFiles folder, showing the Building
object and its parameters.
Building,
Simple One Zone (Wireframe DXF), !- Name
0, !- North Axis {deg}
Suburbs, !- Terrain
0.04, !- Loads Convergence Tolerance Value {W}
0.004, !- Temperature Convergence Tolerance Value {deltaC}
MinimalShadowing, !- Solar Distribution
30, !- Maximum Number of Warmup Days
6; !- Minimum Number of Warmup Days
What are epJSON files?
epJSON files are also text files but are structured according to the widely-used standard JSON format (see https://www.json.org/json-en.html). This means that most programming languages will have built-in parsers for working with JSON files, and by extension epJSON files. In an epJSON file the input objects are presented in a similar manner to an idf file and contain the same information. Below is an example of the same Building object as shown before, but this time converted to epJSON format.
"Building": {
"Simple One Zone (Wireframe DXF)": {
"loads_convergence_tolerance_value": 0.04,
"maximum_number_of_warmup_days": 30,
"minimum_number_of_warmup_days": 6,
"north_axis": 0,
"solar_distribution": "MinimalShadowing",
"temperature_convergence_tolerance_value": 0.004,
"terrain": "Suburbs"
}
If you have an idf file and wish to convert it to an epJSON file, this can be done by using the -c
or --convert
flag of the command line options. See this post for more details on using the command line with EnergyPlus.
1. Using a text editor
As both idf and epJSON files are text files, they can be edited with any text editor. Open the file using a text editor (such as Notepad), make the changes required, and save the file.
The new input file can be used in a EnergyPlus simulation. If there are any errors in the modified file, these will be flagged up in the .err file which one of the results files created following an EnergyPlus simulation.
What are valid changes and additions that can be made to an EnergyPlus input file? The 'Energy+.idd' and 'Energy+.schema.epJSON' files, both found in the EnergyPlus install directory, provide schemas for the idf and epJSON file formats. The meaning behind the various input objects and parameters can be found in the 'Input Output Reference', most conveniently searched on the bigladdersoftware website here but also available as a large pdf document on the EnergyPlus website here.
2. Using the IDF Editor
EnergyPlus ships with a number of utility programmes. The 'IDF Editor' is a simple editing tool for idf files and can be opened from the Windows Start Menu or within the 'PreProcess' folder in the EnergyPlus install directory. Using this software an idf file can be opened, changes can be made manually and the modified file can be saved.
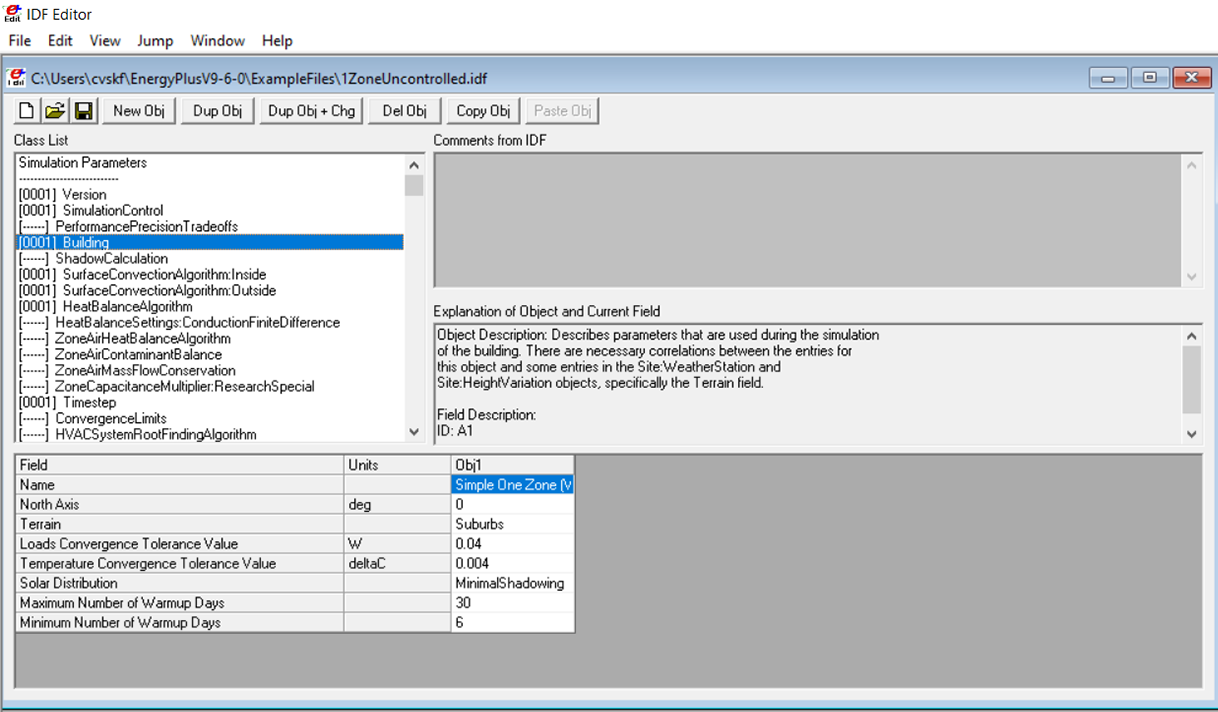
3. Using a JSON editor (epJSON only)
epJSON files are standard JSON files which are structured according to the 'Energy+.schema.epJSON' schema. As a standard file format, which is used widely for sharing information on the internet, these JSON files can be opened and understood by a wide variety of editors.
I use the JSON Editor Online chrome extension which is a Chrome app of the JSON Editor Online website. This provides a useful method of navigating the JSON files through expanding and collapsing the various JSON objects, as shown in the right-hand pane below. Similar to before, this allows the epJSON object to be opened, changes to be made manually and the modified file to be saved.
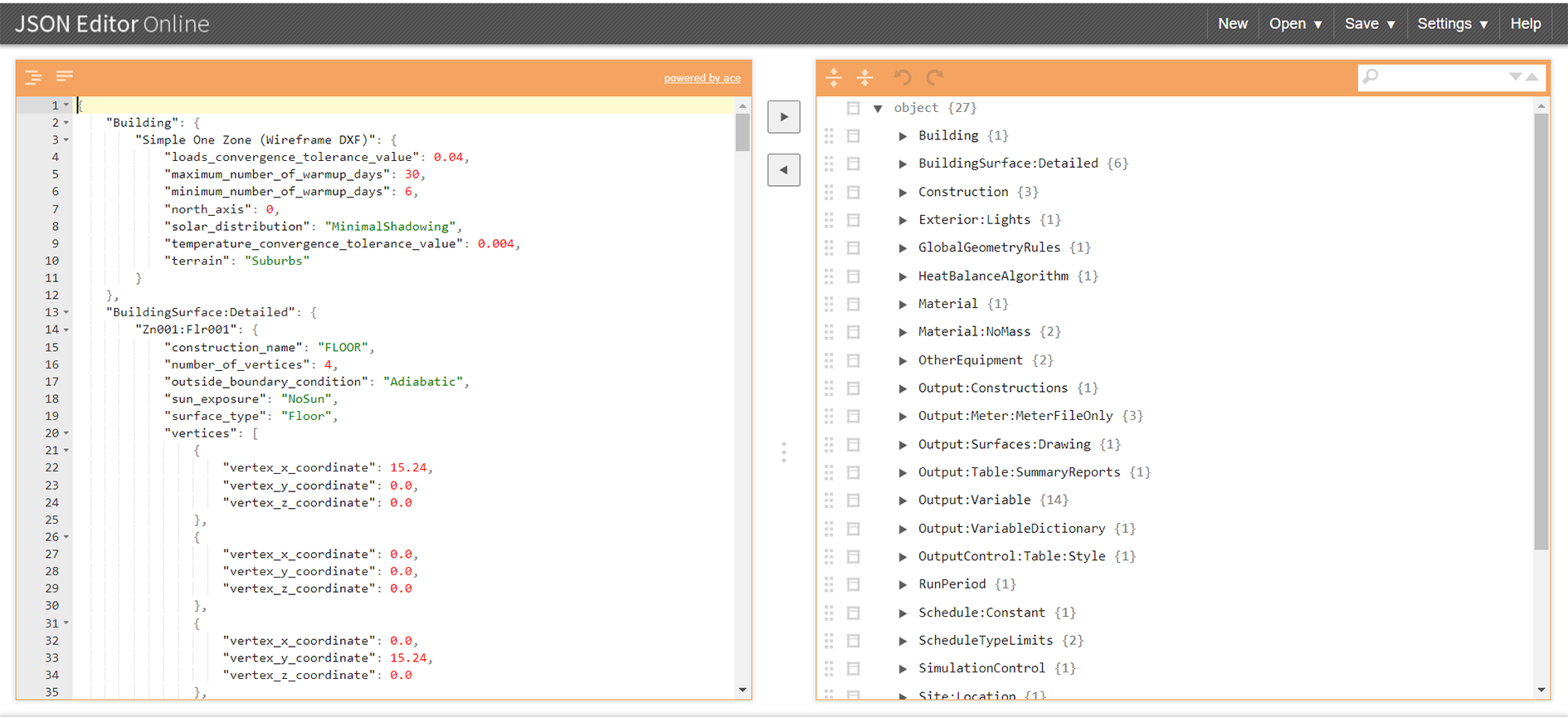
4. Using a custom idf parser (idf only)
The first three options in this list allow us to make manual changes to EnergyPlus input files. But what if we wish to use a programming script, such as Python, to create hundreds or thousands of new idf input files. In such cases we would need to be able to parse (i.e. read in) the idf file using the programming script which would then allow the changes to be made using further programming code.
As the idf file format is a non-standard format unique to EnergyPlus, there won't be any suitable parsers in the standard library of a programming language. The choice is either to make your own or to use one already developed by someone else.
To make your own, the idf is really a simple list data structure - where each item in the list is an individual input object (i.e. 'Building') which in turn is a list itself containing the object parameter (or field) values. It is possible to write the code to read an idf file and place all the data in this list-of-lists structure which can then be modified as needed.
Alternatively there are some 3rd party idf parsers which have already been developed. The most well know of these, at least for Python, is probably eppy which can be used to read, modify and save idf files.
5. Using a JSON parser (epJSON only)
In general it is much easier to work with the epJSON file format than the idf file format, as JSON is a well-known standard for which many editors and parsers have been created.
Python has a JSON parser which comes as part of its standard library, available as the json
package https://docs.python.org/3/library/json.html.
To edit an epJSON file using Python, we would parse the JSON file using the json.load
method. This reads in the data in the JSON file as a nested series of Python dictionaries, lists and other types which can then be edited. The example below shows the Python code required to change the building north_axis parameter from 0 (North) to 90 (East) for the sample Building object.
import json
with open('1ZoneUncontrolled.epJSON') as f:
input_data=json.load(f)
input_data['Building']['Simple One Zone (Wireframe DXF)']['north_axis']=90
with open('new_edit.epJSON','w') as f:
json.dump(input_data,f, indent=4)
A Jupyter notebook with full details of this process is available to view here.
Summary
- There are many different ways to edit EnergyPlus input files.
- I would recommend working with the epJSON input files as there are many existing JSON editors and parsers available.
- For small tests, epJSON files can be edited manually using a JSON editor.
- For larger studies, epJSON files can be edited using prgramming scripts and an associated JSON parser (such as Python and the
json
package in the standard library).