Using Python with IES-VE
This post looks at how to start using Python with the building simulation software IES-VE.
IES-VE is a popular building simulation software tool used throughout the world for building design and regulations compliance. IES is a commercial tool, requiring a paid license. It is possible to drive many aspects of the software using text-based commands in the form of Python scripts. This allows us both to automate the software and to provide some reproducible descriptions of the analysis carried out.
I don't find the Python option in IES-VE particularly intuitive or easy to use, so to explain it I will give a step-by-step tutorial below. This certainly won't explain everything but should give enough information to get started with this.
This tutorial was written using IES Virtual Environment 2012 Feature Pack 4, Version 2021.4.0.0.
Step 1: Create a new IES project
Open the IES software and create a new project using the Small Office starter geometry: New Project -> Create From Starter Geometry -> Small Office
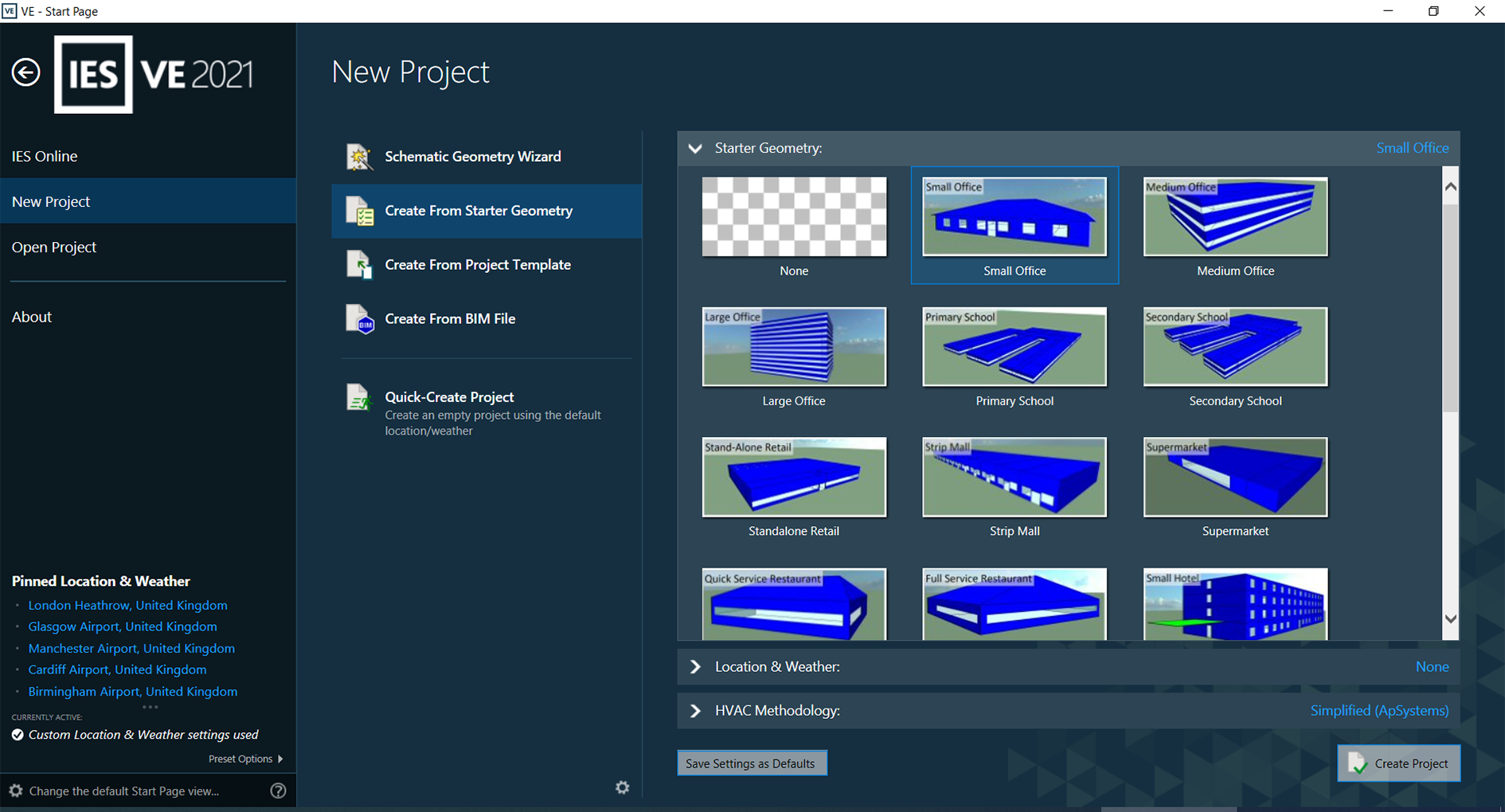
You should now see the Small Office building in the main view of the IES software:
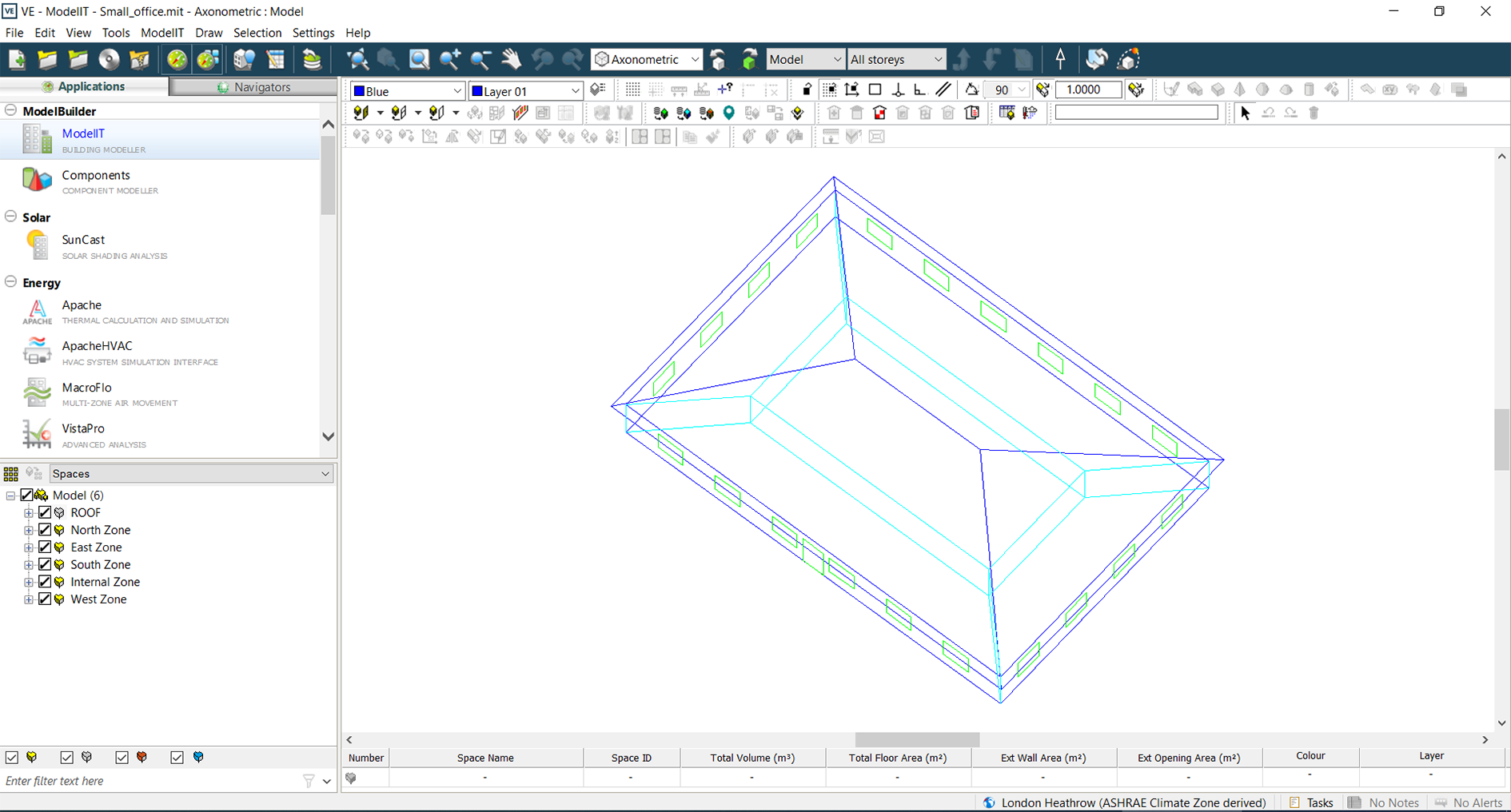
Step 2: Save the project
Save this project to a local directory.
Step 3: Open the VEScripts window
From the top menu choose: Tools -> VEScripts...
(this will only be available if you have purchased the license for the VEScripts option).
You should now see the VEScripts window:
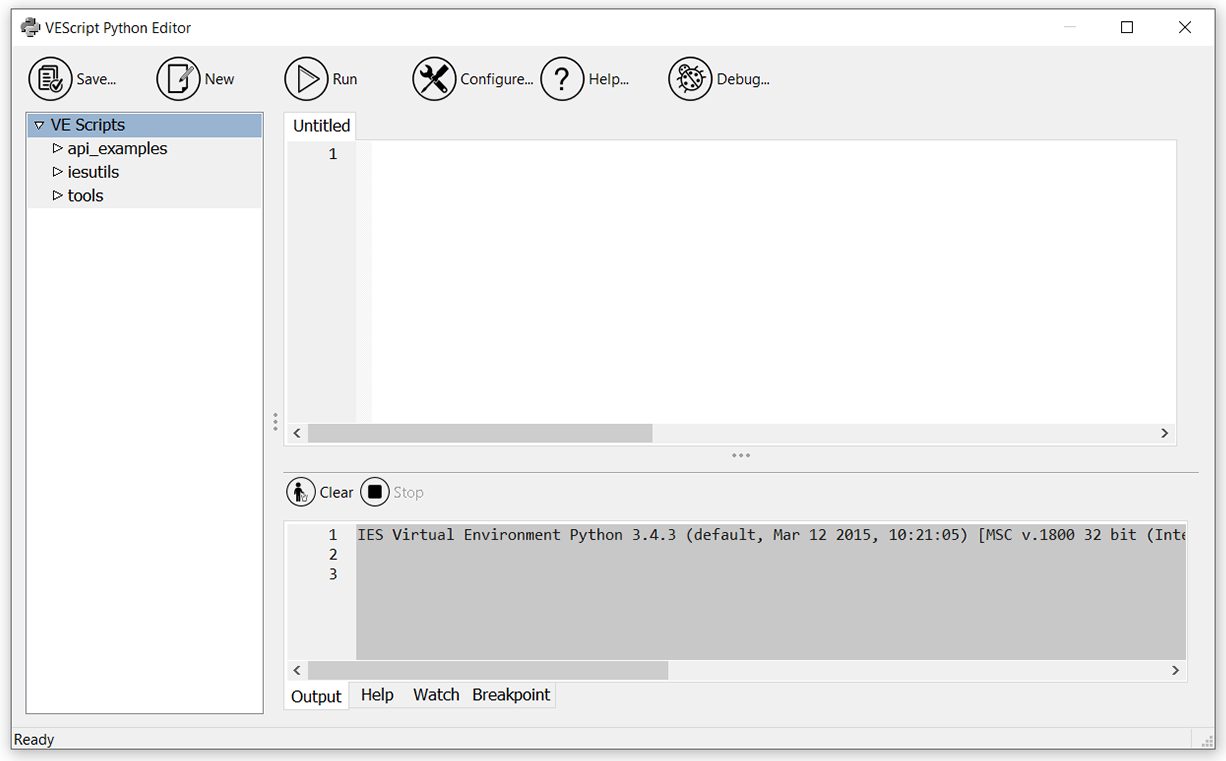
This is a dedicated Python editor that lives 'inside' of the IES-VE software. Python scripts are written and executed here. If you wish to write Python code that interacts with the IES building model or results files, then it has to be written and run in this window.
If, like me, you have your own favourite Python editors (in my case Spyder and Jupyter Notebooks) then this is somewhat of a pain as it's a new interface to learn. A selection of 3rd party libraries are also installed and available to use, including pandas and matplotlib (see the online help for details here). However these may be older versions than you are used to working with.
What can we do with the Python scripting in IES? It seems that it is possible to:
- access the information in the building model, such as the floor areas, construction materials, internal gains etc. This information could be exported for reporting.
- run a simulation and create a new results file.
- edit some details of the model, such as the construction and profiles (not everything can be changed however, it depends on what has been allowed by the API). This could be useful for running multiple simulation of variants on a base case model.
- access the data in a result file. This information could also be exported for reporting, say in a csv file.
Not everything is possible though. In particular it seems that there is little or no access to the model geometry. So there is no way to create new spaces, move spaces, edit the coordinates of spaces etc.
Step 4: Create a local directory for your scripts
Before writing any scripts, we need a place to save them on the local PC. Create a new local folder and then add this to the VEScripts window by:
- right clicking in the 'file list' (the box on the left-hand side where it says 'VEScripts', 'api_examples' etc.)
- choose the
Add script folder
option, and select your newly-created local folder.
Step 5: Create and run a test Python script
Let's make a simple 'Hello world' Python script and run it in the VEScripts editor.
- add a new script
- save it with the name 'test1.py' in your scripts folder created in Step 4 above.
- write the Python code
print('hello')
- run the script
You should see something like this, with the result of the script (the string 'hello' printed out) in the bottom pane:
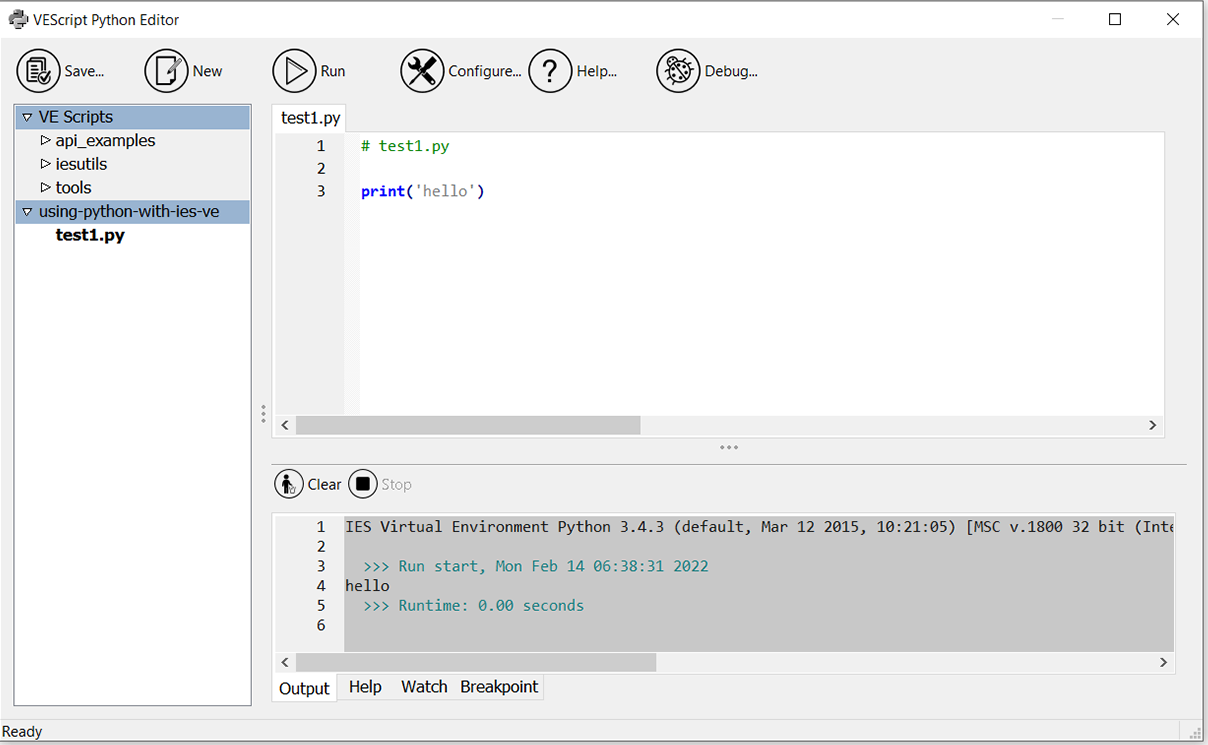
Step 6: Create a Python script that uses the VE Python API
What is the 'VE Python API'? An API is an Application Programming Interface - essentially a way of one programme talking to another programme. In this case the VE Python API allows us to access and manipulate the building model stored in the IES software. It is described in detail in the online help here and can be accessed using the import iesve
command.
Here is an example which lists the rooms (but not the roof void) in the Small Office IES model:
# test2_list_rooms.py
import iesve
project = iesve.VEProject.get_current_project()
models = project.models
realmodel = models[0]
bodies = realmodel.get_bodies(False)
rooms = [body for body in bodies if body.subtype==iesve.VEBody_subtype.room]
for room in rooms:
print(room.id,room.name)
The output of this script is:
>>> Runtime: 0.00 seconds
>>> Run start, Mon Feb 14 07:07:19 2022
NR000000 North Zone
ST000000 East Zone
ST000001 South Zone
NT000000 Internal Zone
WS000000 West Zone
>>> Runtime: 0.01 seconds
You can try this yourself by creating a new blank script in the VEScripts window, copying the Python code above and running the script. You should see the same output.
How does this work? There's a lot of detail here but essentially the script uses the classes and methods exposed by the VE Python API (accessed using the import iesve
line) to query the building model information stored in the IES-VE software. The full details of how these classes and methods work is explained in the online help although, for me at least, it took a number of experiments to see exactly how this all worked. I also found it useful to use the built-in Python dir
command to see the methods and attributes available for the different iesve
classes.
Next steps
- Look at the scripts used in this post on GitHub here.
- Read the online help for the VE Python API here.
- Look at the example scripts which come pre-installed in VE Scripts window, including the folders 'api_examples', 'iesutils' and 'tools'.
- Experiment with your own Python scripts to explore the VE Python API.
- View the methods and attributes of the VE Python API classes using the
dir
built-in function.